Today we will talk about React Native, an application that has gained popularity to develop mobile applications with high quality in a friendly and accessible way for everyone.
If you are thinking about developing an application, you have surely heard that there is the option of developing it with native code or with some technology. React Native is a middle ground between the two, as it is a semi-native technology that has many benefits, including time and cost savings.
Difference between native programming and react native
The main difference between native programming and React Native is that, for the former, a specific code must be written for each platform on which the application is intended to be published, so there must be a specific language for iOS (Swift and Xcode) and another specific for Android (Java and Android Studio).
While with React this is not necessary, with it you can use a single programming language (Java Script) that works on both iOS and Android, making development faster and more efficient.
React Native features
Cross-platform
React is a cross-platform application, due to the fact that it allows you to create mobile apps for iOS and Android and run them on different platforms.
Performance
In terms of speed, the apps produced with this application have a similar one to native apps.
Hot reloading
React has a feature that allows developers to make changes to their application in real time without having to restart the application or stop it every time the source code is modified. So the changes are reflected immediately.
How to enable it?
To enable it, the following code must be added in the index.js or App.js file

Once enabled, developers can execute changes in the source code of their application, save the changes and see the changes in real time in the application without having to restart the application.
It is very important to note that it can present problems such as loss of application state or data corruption at runtime, so you should test the changes very carefully before implementing it in the final application.
Active community
React has a large community of developers, companies and organizations working on developing and improving the platform.
Some of the online platforms where you can find them are: GitHub, Stack Overflow, Reddit, Twitter, among others. There you can get answers to your questions, share knowledge and collaborate on open source projects. These groups organize events, meetings and conferences where developers can meet each other, share their experiences and collaborate on projects.
Third-party support
Because there is such a large community in React, there is also a wealth of third-party libraries and tools that make it easy to create applications. These support providers may include:
Third-party libraries and components: which you'll be able to use to add additional functionality to your mobile apps. These libraries and components can be used to perform tasks such as integration with third-party platforms, payment management, data visualization, among others.
Cloud services: Many cloud service providers offer support for React Native, allowing developers to make use of their services in their mobile apps. They may include cloud storage, user authentication, data analytics, among others.
Development tools: can help developers improve the efficiency and quality of their development processes. These tools can include debuggers, code generators, continuous integration, among others.
Agile development
This application allows developers to adopt agile software development methodologies. These methodologies emphasize continuous delivery of functional and valuable software, collaboration among team members, and rapid response to changes in project requirements.
Some examples of how it can be used in this methodology are:
Continuous delivery: allows developers to make changes to the application and see the results immediately. So it facilitates the continuous delivery of functional and important software to the customer.
Continuous integration: it can automate the process of building, testing and delivering software. It helps reduce errors and speeds up the delivery process, which is a key practice of agile methodology.
Team development: several developers can work on different parts of the application simultaneously, encouraging teamwork.
Flexibility in project requirements: allows developers to quickly execute changes to the application, making it easier to respond to changes in project requirements.
React Native Elements
Components
Within React Native you can use components, and if you are not familiar with programming, the term will be a bit abstract. Components are part of the user interface (UI), they encapsulate functionality and visual elements. They are the basic building block of an application. There are two types of them, class and function.
Function components
- Stylesheet: with which we will add the styles.
- View: this is the most basic component on which most of the other components inherit.
- Text: used to display the texts.
- Image: used to display images.
- TextInput: provides a space for the user to enter text from his device.
- ScrollView: allows you to encompass various components and have users scroll the screen to view them.
Text function component
The basic text component can be programmed in this way:
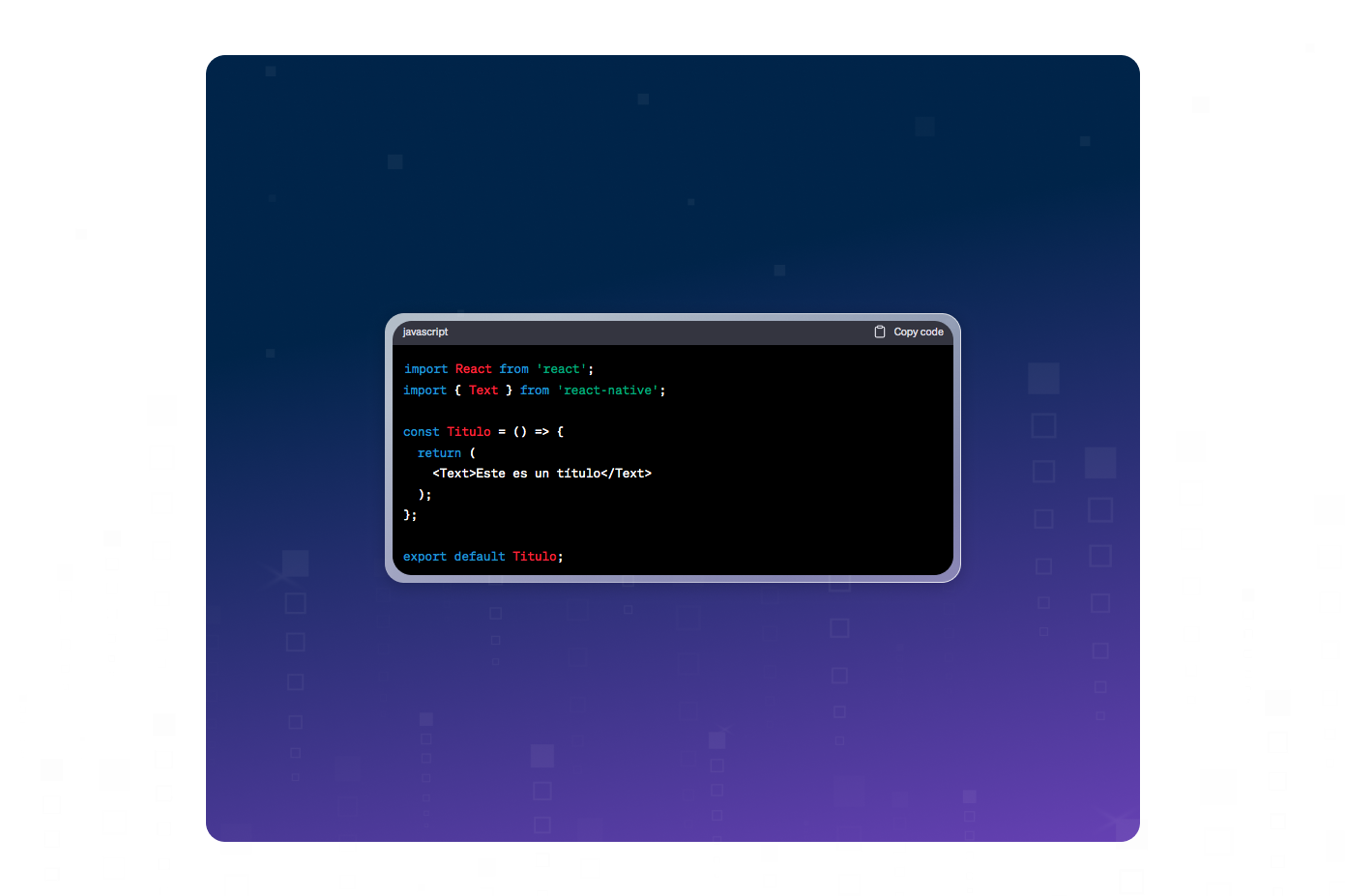
There is also a custom text component in which you can choose the thickness, color, and font of the text. In this example you can see how to style the subtitle component.
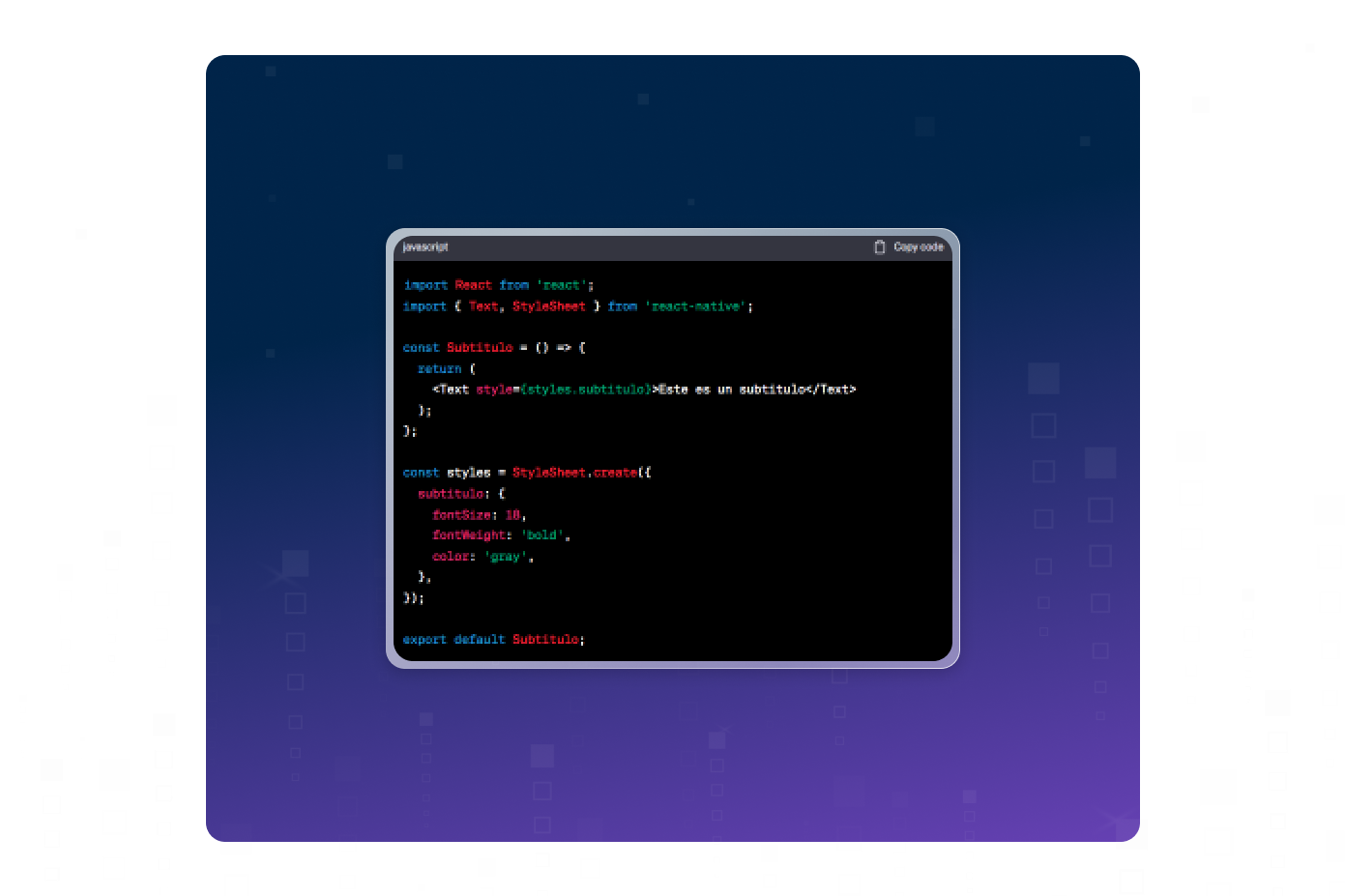
You also have the opportunity to create custom messages in which the user's name is included, to generate the variable the name is shown between braces {} as shown in this image:
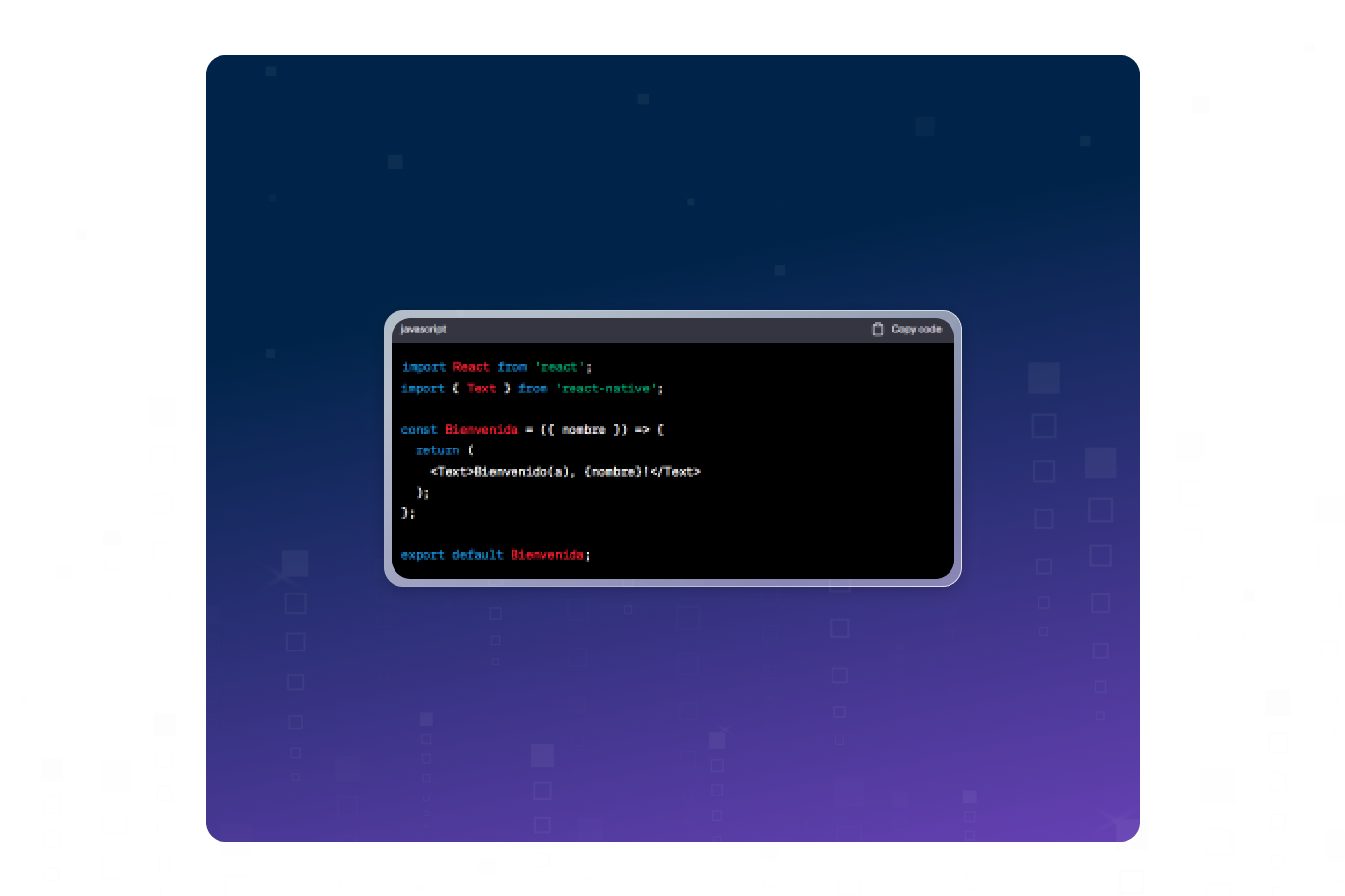
Style function component.
The StyleSheet component is used to define the styles of the components used by your components. To use it you need to import it from the react-native library:
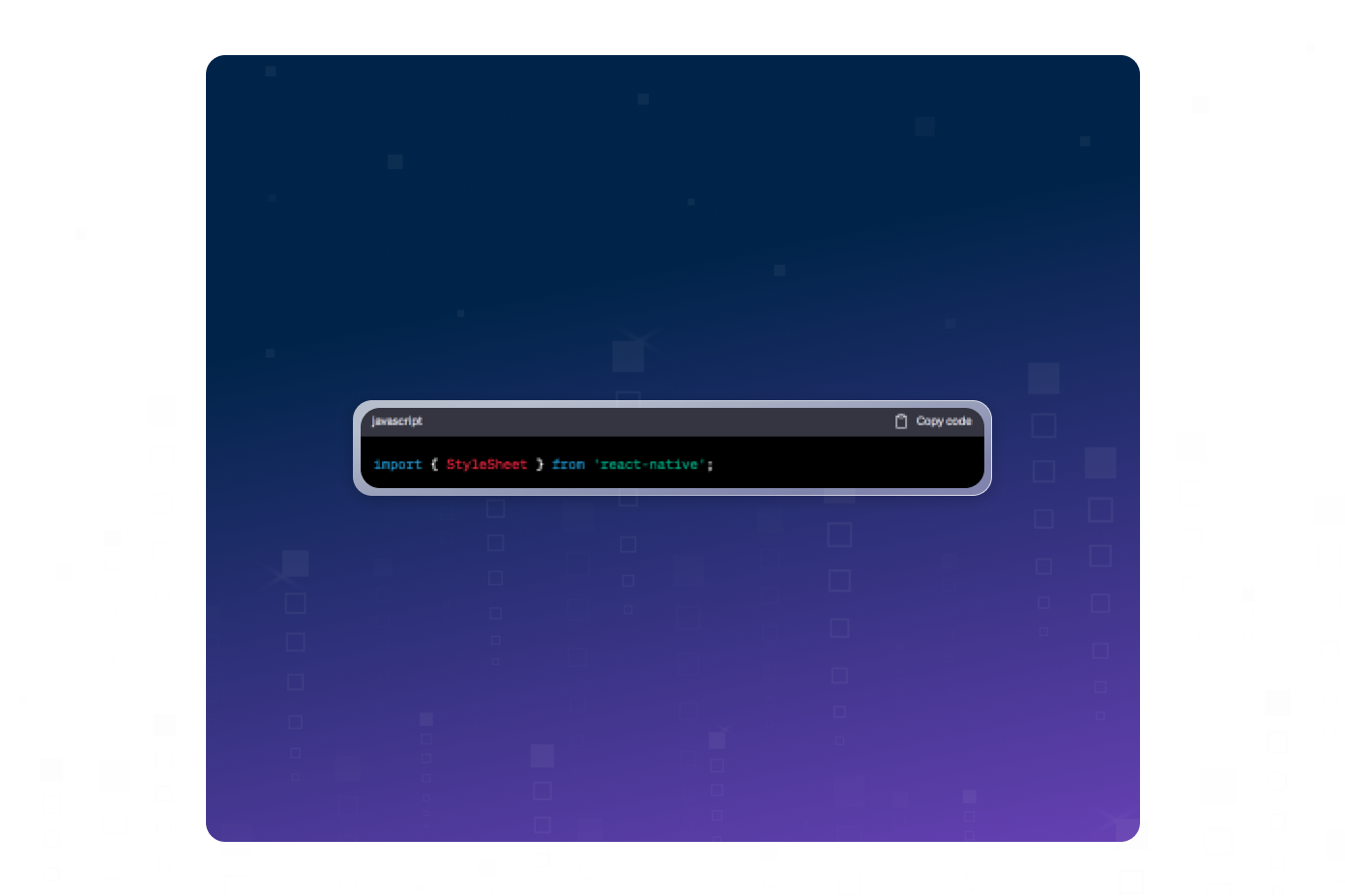
You can then define the styles using the StyleSheet.create() method, which takes an object as an argument, where each key is the name of a style and each value is an object with the properties of the style, as shown below:
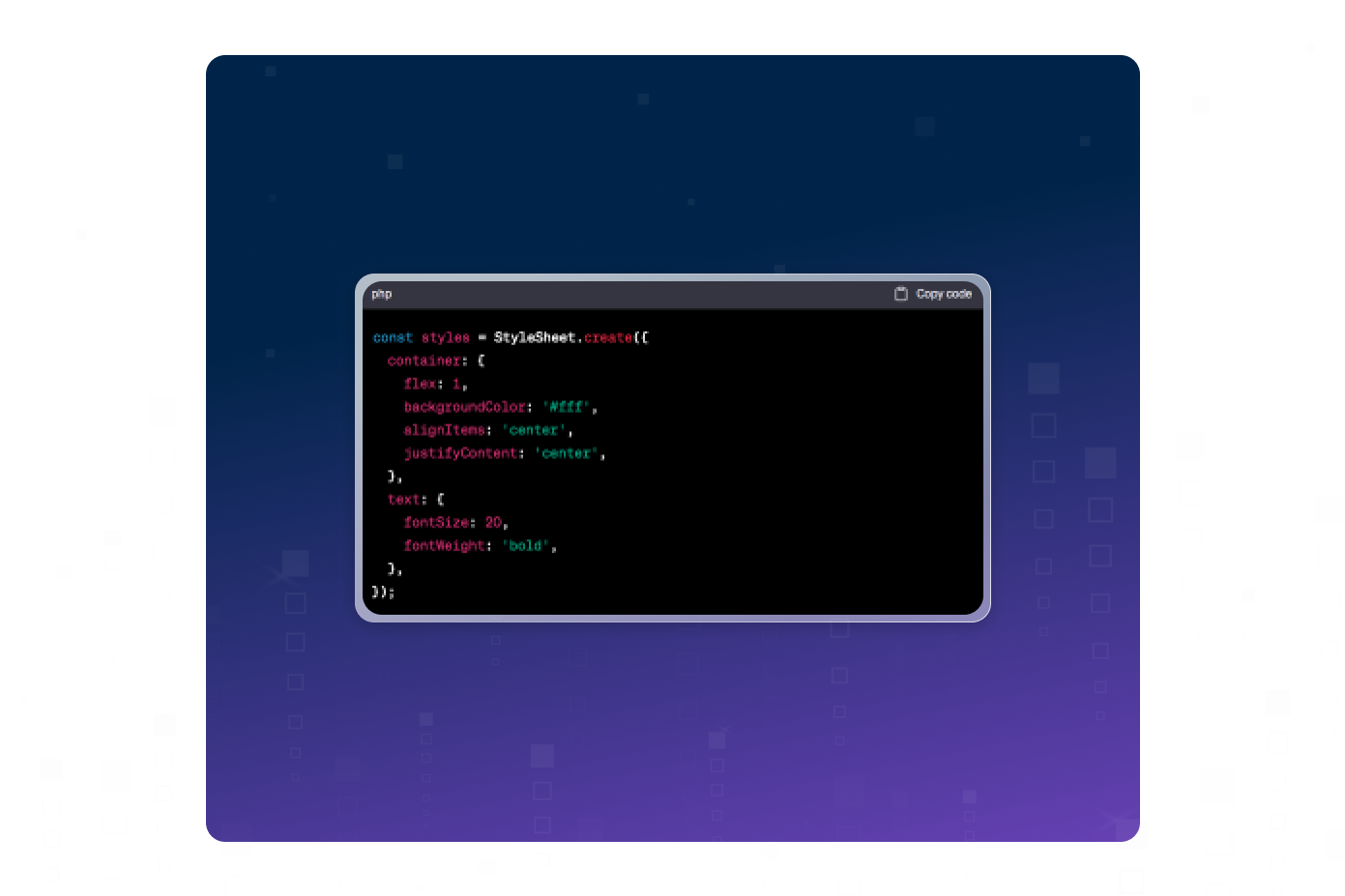
In the above example, two styles were defined: container and text.
The container style sets the flex property to 1, sets the background color to white and centers its content horizontally and vertically.
The text style sets the font size to 20 and the font weight to bold.
To use these styles in your components, you can pass the style object to the style prop property as shown below:

This example shows how the Styles object has been imported from a separate file and was used to apply styles to a View component and a Text component. The View component will have the container style applied to it, and the Text component will have the text style applied to it.
View Function Component
Used to create containers or components that act as building blocks for the user interface. It helps you to define the layout, style and structure of your application as we can see below:
In the previous example, the "View" component was used as a container for other components. Some styles were also defined in the "View" component, such as "flex", "justifyContent" and "alignItems". These styles are used to define how the components are distributed and aligned within the container.
Scrollview function component
Generates a scrollview that allows users to scroll through content that is contained within the ScrollView. This is particularly useful for long content or when screen space is limited. You can use it as shown below:
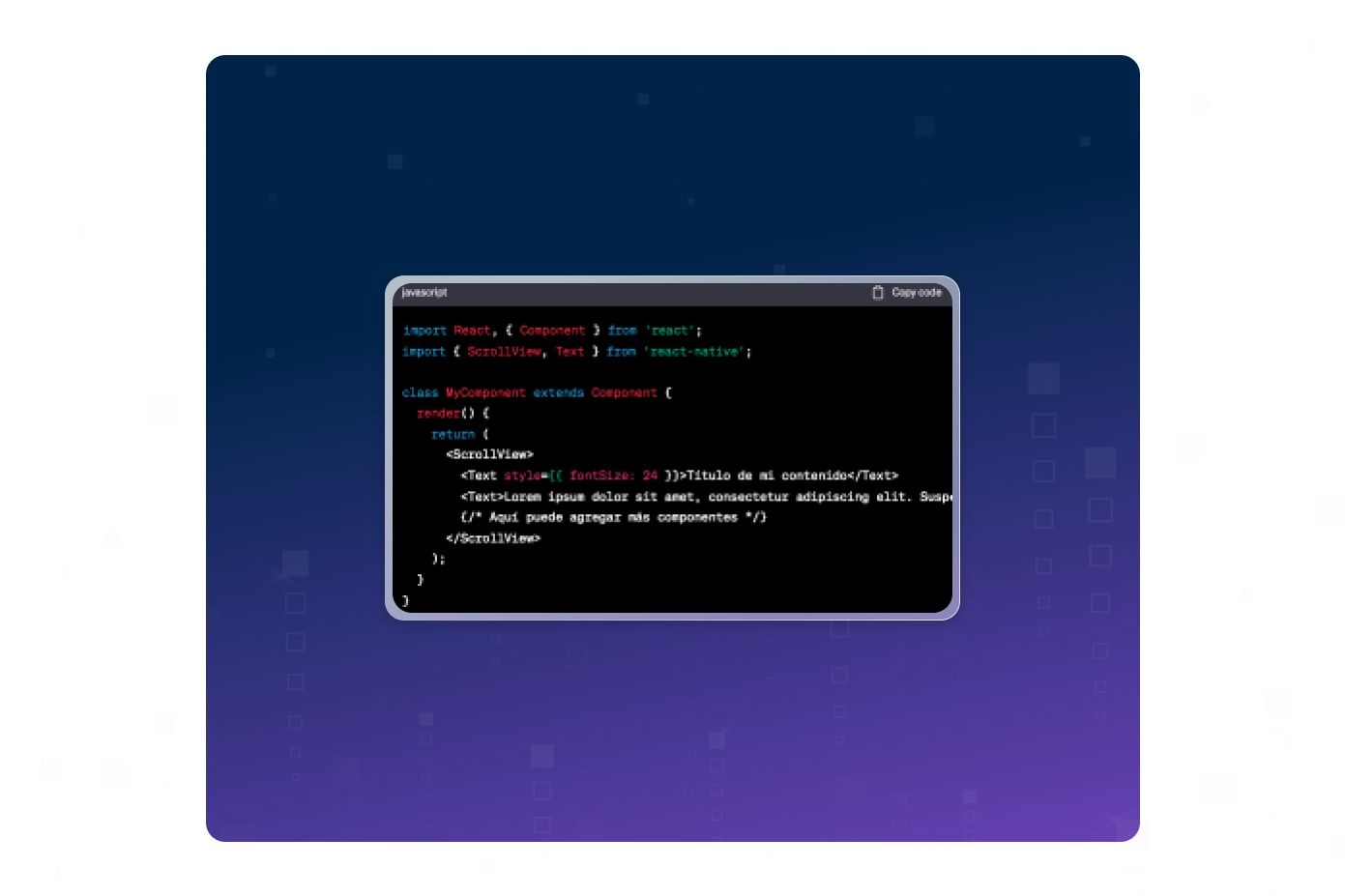
In this example, the ScrollView allows the user to scroll through the content. By having a larger amount of content than the height of the screen, it allows the user to scroll up and down to see all the content.
Image function component
This component is very useful for displaying logos, icons and any other type of image you want to include in your application.
You can use it in this way:
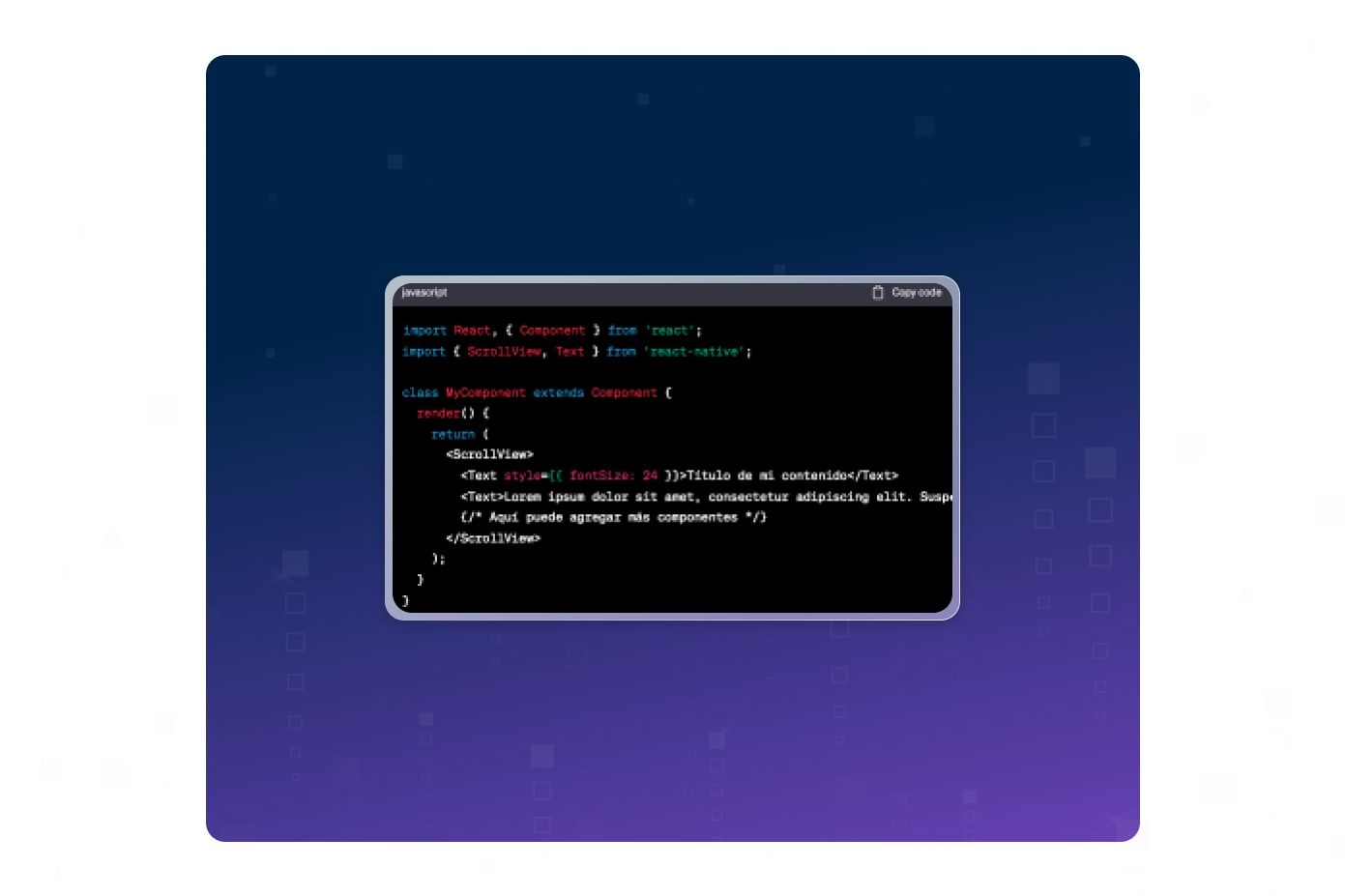
Here the component was used to display an image in the user interface of an application. The "source" property to specify the location of the image we want to display and the "style" property to define its size.
The image will be resized to the dimensions specified in the "style" property if necessary, but will keep its original aspect ratio.
You can use the "resizeMode" option to specify how the image is resized if its size differs from the dimension specified in the style. The "resizeMode" options include "cover", "contain", "stretch", "center" and "repeat".
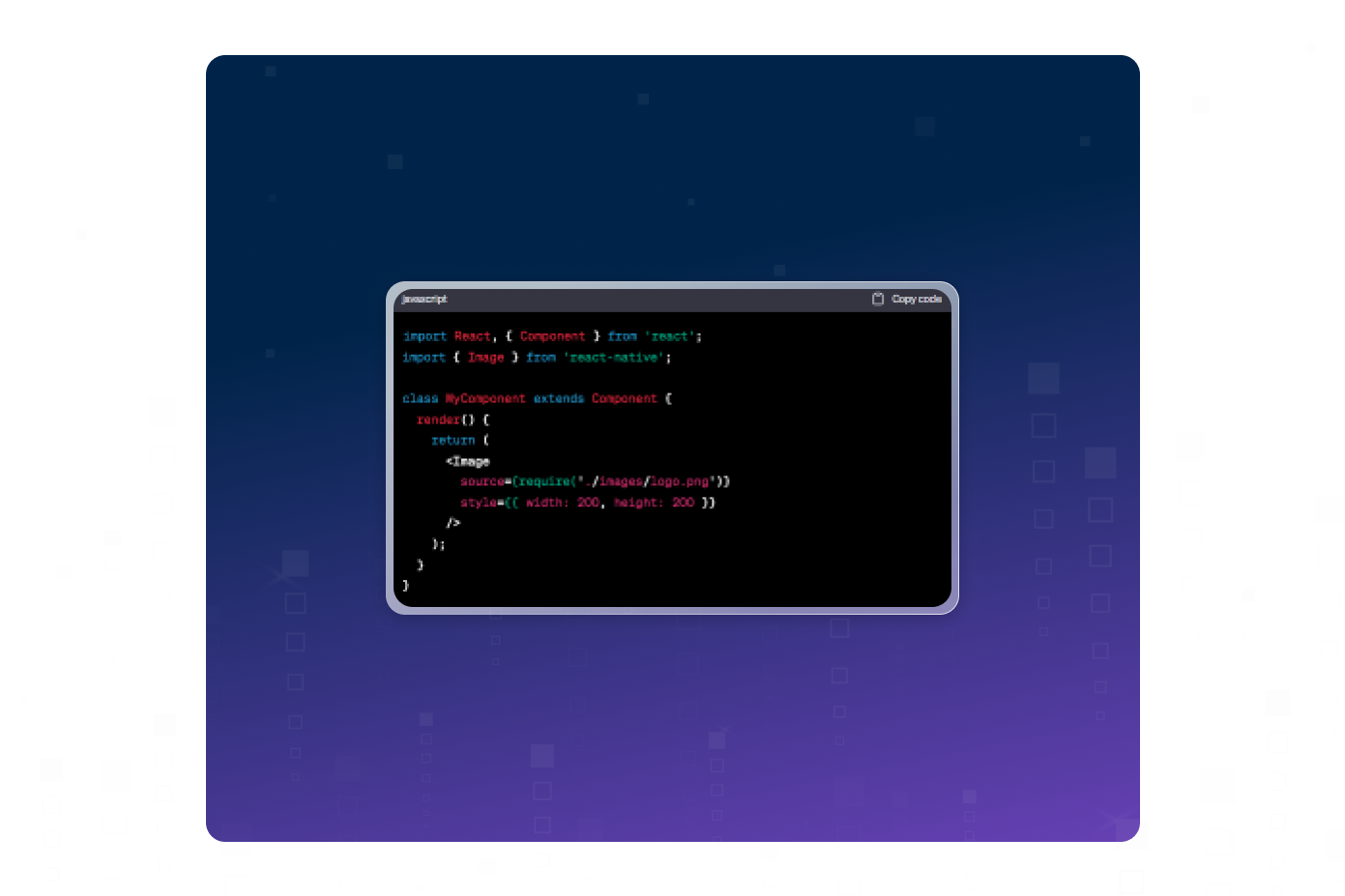
Here the "resizeMode" property with the value "contain" has been used so that the image fits completely within the size specified in the style, maintaining its original aspect ratio.
Textinput function component
Used to create text input fields in the user interface of your application. This component is very useful for allowing users to enter text in your application, such as in registration or search forms. You can use it in this way:
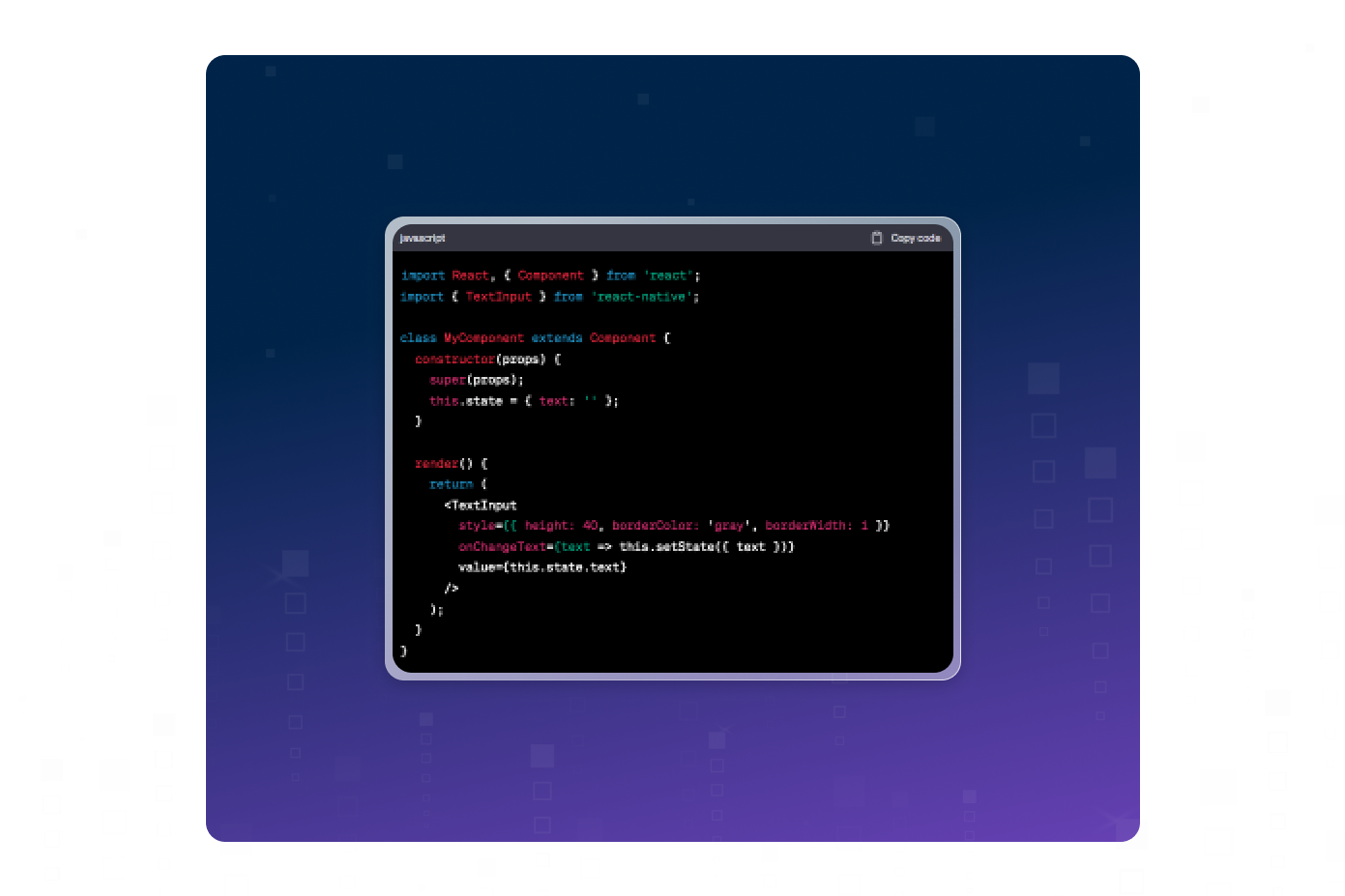
Here it has been used to produce a text input field in the user interface of our application. The "style" component is used to define the style of the text input field and the "onChangeText" property is used to update the state of the component each time the user enters text. The "value" property is used to set the value of the text input field.
You can use other attributes, such as "placeholder" to display a default text in the text input field before the user enters text, "maxLength" to set the maximum allowed length of text that can be entered, "keyboardType" to specify the type of keyboard that is displayed (e.g. numeric, email, etc.), and "keyboardType" to specify the type of keyboard that is displayed, e.g. numeric, email, etc.
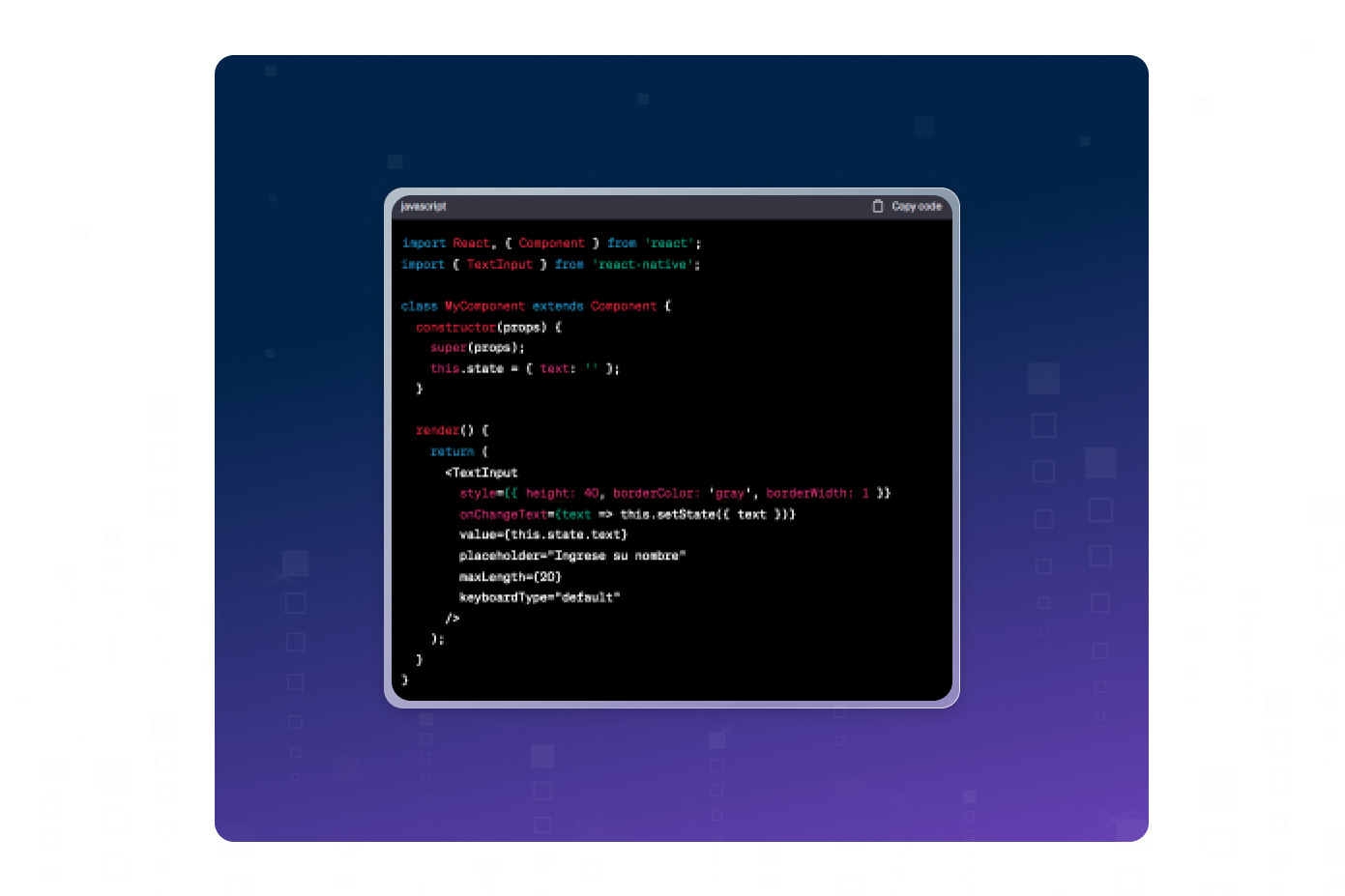
In the example above, we have used several additional properties to further customize our text input field, including "placeholder" to display a default text, "maxLength" to limit the number of characters that can be entered and "keyboardType" to specify the type of keyboard that is displayed on the screen.
This is just a small introduction to the world of React Native and everything you can do in it with the help of the components I mentioned above. No doubt you have everything you need at your fingertips to produce an application and this guide should be more than enough for you to start exploring the React universe.
At BluePixel we also develop applications with React Native, so if you prefer a group of experts to do it for you, don't hesitate to contact us at hola@bluepixel.mx.
If you want to know more about similar topics, keep an eye on our social networks, on Facebook, Instagram and LinkedIn.